Selection sort use case.
The idea behind selection sort algorithm is very simple, we select the smallest element from an any unsorted list in each iteration and place that element at the beginning of the list. It is also called an in-place comparison sorting algorithm. Selection sort is famous for its simplicity and has performance advantages over more complicated algorithms in certain situations, but often inefficient on large lists.
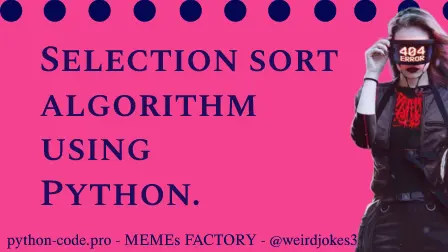
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-02 by Andrey BRATUS, Senior Data Analyst.
Selection sort Python code:
Selection sort code result:
The algorithm deals with two subarrays in a given array.
New subarray which is already sorted.
Old remaining subarray which is unsorted.
In every iteration of selection sort, the minimum element (for ascending order) from the unsorted list is picked and moved to the sorted list.
def selection_Sort(array, size):
for step in range(size):
min_idx = step
for i in range(step + 1, size):
# to change to descending order, change > to < in this line
# select the min element in each iteration
if array[i] < array[min_idx]:
min_idx = i
# put min at the correct position
(array[step], array[min_idx]) = (array[min_idx], array[step])
data = [-10, 35, 0, 13, -7]
size = len(data)
selection_Sort(data, size)
print('Sorted elements in ascending order::')
print(data)
OUT: Sorted elements in ascending order:
[-10, -7, 0, 13, 35]