Double-ended queue use case.
In computer science and programming a deque or a double-ended queue is an abstract data type that defines a queue, for which items can be added to or removed from either the front (head) or back (tail). It is also often referred as a head-tail linked list.
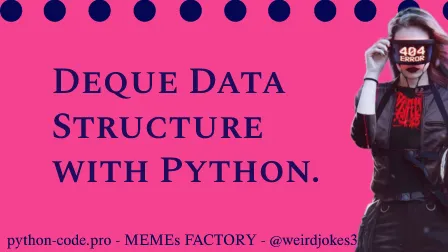
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Deque Data Structure Python code:
Deque Data Structure Python code output:
The double-ended queue can be implemented in many different ways using Python, but the most convinient way is to use collections module.
The following operations/methods are supported by deque:
append():- to insert the value in its argument to the right end of the deque.
appendleft():- to insert the value in its argument to the left end of the deque.
pop():- to delete an argument from the right end of the deque.
popleft():- to delete an argument from the left end of the deque.
from collections import deque
# initializing deque
de = deque([1,1,1])
print ("Created deque : ")
print (de)
# insert element at right end
de.append(2)
print ("Appending at right : ")
print (de)
# insert element at right end
de.append(3)
print ("Appending at right : ")
print (de)
# insert element at left end
de.appendleft(5)
print ("Appending at left : ")
print (de)
# insert element at left end
de.appendleft(7)
print ("Appending at left : ")
print (de)
# delete element from right end
de.pop()
print ("Deleting from right : ")
print (de)
# delete element from left end
de.popleft()
print ("Deleting from left: ")
print (de)
OUT:
Created deque :
deque([1, 1, 1])
Appending at right :
deque([1, 1, 1, 2])
Appending at right :
deque([1, 1, 1, 2, 3])
Appending at left :
deque([5, 1, 1, 1, 2, 3])
Appending at left :
deque([7, 5, 1, 1, 1, 2, 3])
Deleting from right :
deque([7, 5, 1, 1, 1, 2])
Deleting from left:
deque([5, 1, 1, 1, 2])