Leap Year detection use case.
A leap year (intercalary year) is a calendar year that contains an additional day that should be added to keep the calendar year synchronized with the astronomical year.
In the most commonly used Gregorian calendar three simple conditions are used to identify leap years:
- The year can be evenly divided by 4, is a leap year, unless:
- The year can be evenly divided by 100, it is NOT a leap year, unless:
- The year is also evenly divisible by 400. Then it is a leap year.
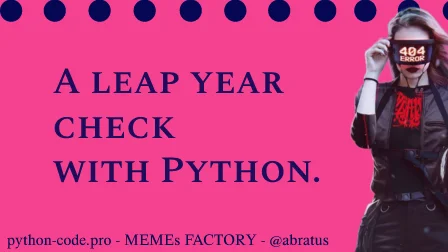
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
A leap year check with Python - classic solution:
A leap year check with Python - using calendar:
A leap year check is the most common Python programming job interview question that tests candidates the ability to handle conditional statements. There are several solutions to this task available as usual in programming, the most obvious and using calendar function.
year = 2022
if (year % 400 == 0) and (year % 100 == 0):
print("{0} is a leap year".format(year))
elif (year % 4 ==0) and (year % 100 != 0):
print("{0} is a leap year".format(year))
else:
print("{0} is not a leap year".format(year))
OUT: 2022 is not a leap year
from calendar import isleap
print(isleap(2022))
OUT: False