Priority queue use case.
In computer science and programming, a priority queue is an abstract data structure similar to a regular queue in which each item additionally has a "priority" assigned to it. In a priority queue, an element with high priority is selected/served before low priority element. If items with the same priority occur, they are selected/served according to their queue order.
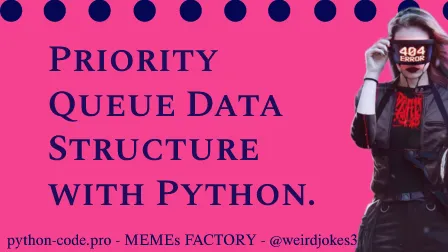
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Priority Queue Data Structure Python code.
Priority Queue Data Structure Python code result.
The FIFO rule is applied in a normal queue, the values are removed on the basis of priority in a priority queue. Usually the value of the element is considered for assigning the priority, the item with the highest value is asigned the highest priority. In some cases, we assign the highest priority to the element with the lowest value.
class PriorityQueue(object):
def __init__(self):
self.queue = []
def __str__(self):
return ' '.join([str(i) for i in self.queue])
# check if the queue is empty
def isEmpty(self):
return len(self.queue) == 0
# inserting an element
def insert(self, data):
self.queue.append(data)
# popping an element
def delete(self):
try:
max_val = 0
for i in range(len(self.queue)):
if self.queue[i] > self.queue[max_val]:
max_val = i
item = self.queue[max_val]
del self.queue[max_val]
return item
except IndexError:
print()
exit()
theQueue = PriorityQueue()
theQueue.insert(1)
theQueue.insert(11)
theQueue.insert(13)
theQueue.insert(3)
theQueue.insert(5)
theQueue.insert(25)
print ("Original queue: ")
print(theQueue)
print ("Removing 3 elements one-by-one: ")
theQueue.delete()
print(theQueue)
theQueue.delete()
print(theQueue)
theQueue.delete()
print(theQueue)
OUT:
Original queue:
1 11 13 3 5 25
Removing 3 elements one-by-one:
1 11 13 3 5
1 11 3 5
1 3 5