Queue as FIFO use case.
In computer science and programming a queue is an abstract data structure - (ADT) which is a collection of items that are maintained in a sequence and can be modified by the addition of elements at one end of the sequence
and the removal of elements from the other end of the sequence.
It is similar to the queue in a supermarket, where the first person entering the queue is the first person who get served.
Thus the queue follows the FIFO principle (First In First Out) - the element that goes in first is the element that comes out first.
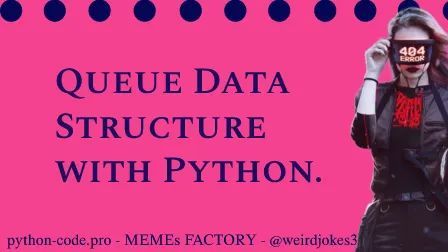
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Queue Data Structure Python code:
Queue Data Structure Python code output:
The Queue has following operations and sptates:
- Enqueue: Add an item to the end.
- Dequeue: Remove an item from the front.
- IsEmpty: Check if the queue is empty.
- IsFull: Check if the queue is full.
- Peek: Get the value of the front of the queue without removing it.
class Queue:
def __init__(self):
self.queue = []
# Adding element
def enqueue(self, item):
self.queue.append(item)
# Removing element
def dequeue(self):
if len(self.queue) < 1:
return None
return self.queue.pop(0)
# Displaying the queue
def display(self):
print(self.queue)
def size(self):
return len(self.queue)
print("Empty queue:")
q = Queue()
q.display()
print("Adding elements:")
q.enqueue(1)
q.enqueue(2)
q.enqueue(3)
q.enqueue(4)
q.enqueue(5)
q.enqueue(6)
q.enqueue(7)
q.enqueue(8)
q.enqueue(9)
q.display()
print("Removing element:")
q.dequeue()
q.display()
print("Removing element:")
q.dequeue()
q.display()
print("Removing element:")
q.dequeue()
q.display()
OUT:
Empty queue:
[]
Adding elements:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Removing element:
[2, 3, 4, 5, 6, 7, 8, 9]
Removing element:
[3, 4, 5, 6, 7, 8, 9]
Removing element:
[4, 5, 6, 7, 8, 9]