Linked list use case.
In computer science and programming, a linked list is a linear collection of data elements whose order is not given by their contiguous physical placement in memory. In a linked list each element points to the next. So it is a data structure consisting of a collection of nodes which together represent a sequence. Each node contains a data and a reference or a link to the next node in the sequence. The last node in the linked list has it's link to NULL.
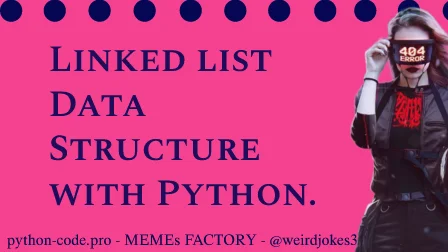
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Linked list Python code:
Linked list code result:
Such structure permits efficient elements insertion or removal operations from any position in the sequence during iteration. Linked Lists in computer science have many use cases starting from stacks, queue and hash tables implementation and dynamic memory handling and version control functionality in programming.
class Node:
def __init__(self, item):
self.item = item
self.next = None
class LinkedList:
def __init__(self):
self.head = None
# Create an instance of linked list
linked_list = LinkedList()
# Set the values
linked_list.head = Node(1)
second = Node(3)
third = Node(5)
fourth = Node(7)
fifth = Node(9)
# Connect nodes
linked_list.head.next = second
second.next = third
third.next = fourth
fourth.next = fifth
# Print the linked list
print("Linked list created :")
while linked_list.head != None:
print(linked_list.head.item, end=" ")
linked_list.head = linked_list.head.next
OUT: Linked list created :
1 3 5 7 9