Sinking sort use case.
Bubble sort (sinking sort) is a data sorting comparison-based algorithm that compares two adjacent elements and swaps them until they are not in the intended order. It is called a bubble sort because it reminds the movement of air bubbles in the water that rise up to the surface, each element of the array moving to the end in each iteration.
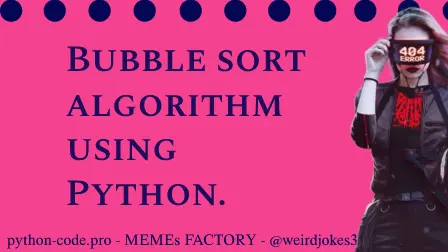
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-09 by Andrey BRATUS, Senior Data Analyst.
Bubble sort Python code:
Bubble sort code output:
The workflow is simple:
Starting from the first item, compare the first and the second elements.
If the first element is greater than the second element, they should be swapped.
Then compare the second and the third elements. Swap items if they are not in order.
The process continues until the last element.
Proceed for the remaining iterations.
def bubbleSort(array):
# loop for each element
for i in range(len(array)):
# loop to compare elements
for j in range(0, len(array) - i - 1):
# compare two adjacent elements
# change > to < to sort in descending order
if array[j] > array[j + 1]:
# swapping elements if they are not in the order
temp = array[j]
array[j] = array[j+1]
array[j+1] = temp
data = [-10, 35, 0, 13, -7]
bubbleSort(data)
print('Sorted elements in ascending order:')
print(data)
OUT: Sorted elements in ascending order:
[-10, -7, 0, 13, 35]