Bin sort use case.
Bucket sort (sometimes called bin sort) is a sorting algorithm that distributes the elements of an original array into a number of buckets or bins. Then each bucket is then sorted individually, either applying a different sorting algorithm, or by recursively using the bucket sort. Finally all sorted buckets are combined to form a final result array.
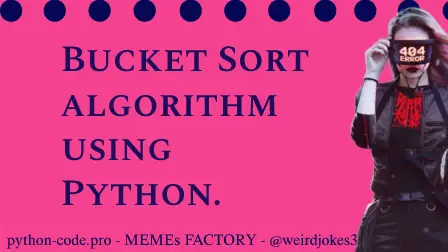
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Bucket Sort Python code:
Bucket Sort Python code output:
Bucket sort is mainly useful when input is uniformly distributed over a range and contains floating points values.
def bucket_Sort(array):
bucket = []
# Empty buckets creation
for i in range(len(array)):
bucket.append([])
# Putting elements into new respective buckets
for j in array:
index_b = int(10 * j)
bucket[index_b].append(j)
# Sorting the elements of each bucket
for i in range(len(array)):
bucket[i] = sorted(bucket[i])
# Getting the sorted elements
k = 0
for i in range(len(array)):
for j in range(len(bucket[i])):
array[k] = bucket[i][j]
k += 1
return array
array = [.44, .32, .33, .11, .22, .47, .55]
print("Sorted elements in ascending order: ")
print(bucket_Sort(array))
OUT: Sorted elements in ascending order:
[0.11, 0.22, 0.32, 0.33, 0.44, 0.47, 0.55]