Radix Sort use case.
Radix or base is the number of unique digits (including zero) used to represent numbers in a positional numeral system.
The concept of Radix Sort is to implement digit by digit sorting beginning from least significant to most significant digit.
Radix sort uses counting sort as a subroutine to sort.
Input array must have the elements with the same radix and width, data must be between a range of elements, it works on sorting based on individual digit position.
Radix is not an in-place algorithm as it uses a temporary count array.
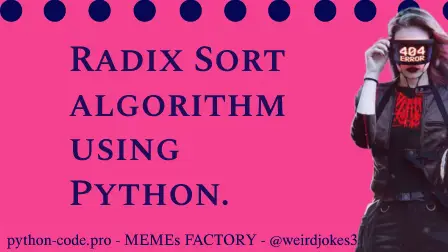
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-12 by Andrey BRATUS, Senior Data Analyst.
Radix Sort Python code:
Radix Sort Python code results:
Since radix sort is a non-comparative algorithm, it has advantages over comparative sorting algorithms.
# counting sort
def countingSort(array, place):
size = len(array)
output = [0] * size
count = [0] * 10
# Calculate count of elements
for i in range(0, size):
index = array[i] // place
count[index % 10] += 1
# Calculate cumulative count
for i in range(1, 10):
count[i] += count[i - 1]
# Place the elements in sorted order
i = size - 1
while i >= 0:
index = array[i] // place
output[count[index % 10] - 1] = array[i]
count[index % 10] -= 1
i -= 1
for i in range(0, size):
array[i] = output[i]
# radix sort - counting sort as subprocess
def radixSort(array):
# find maximum element
max_element = max(array)
# Apply counting sort to sort elements based on place value.
place = 1
while max_element // place > 0:
countingSort(array, place)
place *= 10
data = [1, 3, 2, 5, 7]
radixSort(data)
print("Sorted elements in ascending order: ")
print(data)
OUT: Sorted elements in ascending order:
[1, 2, 3, 5, 7]