Insertion sort use case.
The idea behind insertion sort algorithm is to use cards sorting method as in a bridge card game, we just places an unsorted element at its suitable place in each iteration.
We start supposing that the first card is already sorted then, then we select an unsorted card. If the new card is greater than the card in hand,
we put it on the right, otherwise to the left. In the same way we treat other unsorted cards and put them in their corresponding place.
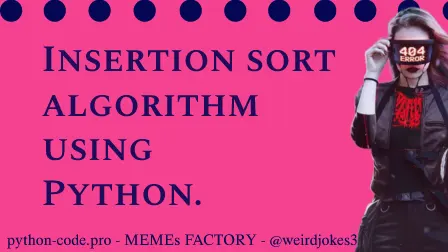
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-13 by Andrey BRATUS, Senior Data Analyst.
Insertion Sort Python code:
Insertion Sort Python code output:
The algorithm implies the sorted list creation based on step-by-step comparison of each element in the list with its adjacent element. Insertion sort is simple in implementation but is much less efficient on large lists than more advanced algorithms (quicksort, heapsort, merge sort...).
def insertion_Sort(list1):
for step in range(1, len(list1)):
elemetnt = list1[step]
j = step - 1
# Compare elemetnt with each element on the left until the smaller element is found
# For descending case, change elemetntlist1[j].
while j >= 0 and elemetnt < list1[j]:
list1[j + 1] = list1[j]
j = j - 1
# Place key at after the element just smaller than it.
list1[j + 1] = elemetnt
data = [-10, 35, 0, 13, -7]
insertion_Sort(data)
print('Sorted elements in ascending order:')
print(data)
OUT: Sorted elements in ascending order:
[-10, -7, 0, 13, 35]