Fizzbuzz use case.
Fizzbuzz is a group word&math game for children to train their division skills.
Rules are very simple.
Players sit in a circle and take turns to count incrementally from 1 to 100, replacing any number divisible by three with the word "fizz", and any number divisible by five with the word "buzz".
Numbers divisible by 15 become "fizzbuzz". A player who makes a mistake is eliminated.
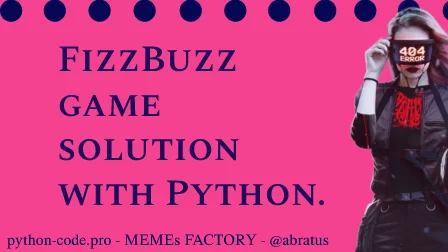
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-10 by Andrey BRATUS, Senior Data Analyst.
Fizzbuzz output with Python - classic solution:
Fizzbuzz output with Python - advanced solution:
FizzBuzz is the most common job interview question in Python computer programming that tests candidates the ability to handle conditional statements. There are several solutions to this task available as usual in programming, the most simple and one of the advanced implementation are below.
for i in range(1, 101):
if i%3==0 and i%5==0:
print('FizzBuzz')
elif i%3==0:
print('Fizz')
elif i%5==0:
print('Buzz')
else:
print(i)
[print(i%3//2*'Fizz'+i%5//4*'Buzz' or i+1) for i in range(100)]