Circular queue use case.
In computer science and programming a circular queue is a data structure that uses a single buffer as if a simple queue were connected end-to-end forming a circle-like structure. In other words it is the extended version of a regular queue where the last element is connected to the first element. The circular buffer works as a FIFO (first in, first out) buffer while a sregular queue buffer works as LIFO (last in, first out) buffer.
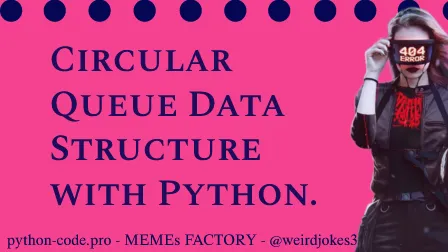
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-05 by Andrey BRATUS, Senior Data Analyst.
Circular Queue Data Structure Python code:
Circular Queue Data Structure Python code result:
The Circular Queue is implemented by the method of circular increment, incrementing the pointer and reaching the end of the queue, we point at the beginning of the queue. It can be implemented in many different ways using Python, but the most convinient way is to use deque from collections module.
from collections import deque
print ("Creating the circular_queue: ")
circular_queue = deque([1,2,3], maxlen=5)
print (circular_queue)
print ("Appending the element: ")
circular_queue.append(4)
print (circular_queue)
print ("Appending the element: ")
circular_queue.append(5)
print (circular_queue)
print ("Extending the element: ")
circular_queue.extend([6])
print (circular_queue)
print ("Popping the element: ")
print(circular_queue.pop())
print (circular_queue)
print ("Rotating the pointer: ")
# rotate the pointer, negative to the left, positive to the right
circular_queue.rotate(-1)
print (circular_queue)
OUT:
Creating the circular_queue:
deque([1, 2, 3], maxlen=5)
Appending the element:
deque([1, 2, 3, 4], maxlen=5)
Appending the element:
deque([1, 2, 3, 4, 5], maxlen=5)
Extending the element:
deque([2, 3, 4, 5, 6], maxlen=5)
Popping the element:
6
deque([2, 3, 4, 5], maxlen=5)
Rotating the pointer:
deque([3, 4, 5, 2], maxlen=5)