Linear Search use case.
In computer science Linear search is the simplest searching algorithm. It is also called a sequential searching algorithm which starts from one end and check every element of the list until the desired element is found. If the algorithm reaches the end of the list, the search terminates unsuccessfully.
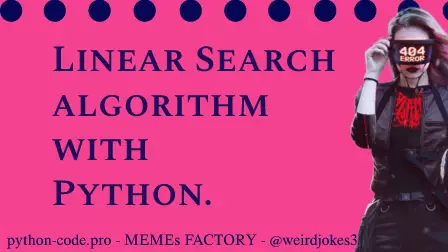
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-03 by Andrey BRATUS, Senior Data Analyst.
Linear Search Python code:
Linear Search code output:
The performance of linear search shows grete results if the desired value is more likely to be near the beginning of the list than to its end. Practically linear search is usually very simple to implement, and is efficient when the list has only a few elements, or when performing a single search in an un-ordered list.
def linear_Search(array, n, x):
# Searching through array sequencially
for i in range(0, n):
if (array[i] == x):
return i
return -1
array = [3, 5, 4, 0, 12, 11]
x = 12
n = len(array)
result = linear_Search(array, n, x)
if(result == -1):
print("Searched element not found.")
else:
print("Searched element found at index: ", result)
OUT: Searched element found at index: 4