Shell's method use case.
Shellsort or Shell's method (named after Donald L. Shell ) is an in-place comparison sort.
It can be described as either a generalization of sorting by exchange e g bubble sort or insertion sort.
The sorting starts from elements that are far apart from each other and successively reduces the interval between the elements to be sorted.
By starting with far apart elements, it can move some wrong placed elements into position faster than a simple nearest neighbor exchange.
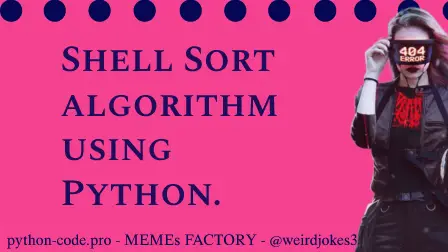
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-25 by Andrey BRATUS, Senior Data Analyst.
Shell Sort Python code:
Shell Sort Python code results:
The performance of the shell sort algorithm depends on the type of sequence used for a given input array. It used mainly for medium to large-sized datasets to reduce the number of operations.
def shell_Sort(array, n):
# Rearranging elements at each n/2, n/4, n/8, ... intervals
interval = n // 2
while interval > 0:
for i in range(interval, n):
temp = array[i]
j = i
while j >= interval and array[j - interval] > temp:
array[j] = array[j - interval]
j -= interval
array[j] = temp
interval //= 2
data = [-10, -7, 0, 13, 35]
size = len(data)
shell_Sort(data, size)
print('Sorted elements in ascending order :')
print(data)
OUT: Sorted elements in ascending order :
[-10, -7, 0, 13, 35]