Bot for Wikipedia.
PAs you know chat bots are specially built software programs that interacts with internet users automatically. Bots can communicate with people from social media or simple websites. Python uses many libraries such as NLTK to implement Natural language Processing (NLP) function (can be considered as AI) which generates replies based on users input.
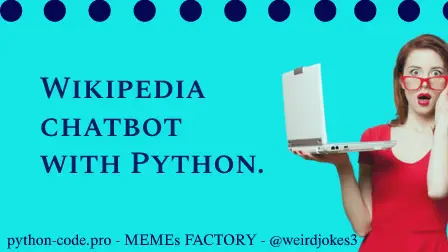
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
An example below uses wikipedia library to seach an answer to users question with a selected topic - 'Woman'. So now you can find out everything about women you always wanted to know !!!
Simple Python Wikipedia chatbot:
import nltk
from nltk.stem import WordNetLemmatizer
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
import wikipedia
nltk.download('averaged_perceptron_tagger')
nltk.download('wordnet')
nltk.download('punkt')
text = wikipedia.page('Woman').content
lemmatizer = WordNetLemmatizer()
def lemma_me(sent):
sentence_tokens = nltk.word_tokenize(sent.lower())
pos_tags = nltk.pos_tag(sentence_tokens)
sentence_lemmas = []
for token, pos_tag in zip(sentence_tokens, pos_tags):
if pos_tag[1][0].lower() in ['n', 'v', 'a', 'r']:
lemma = lemmatizer.lemmatize(token, pos_tag[1][0].lower())
sentence_lemmas.append(lemma)
return sentence_lemmas
def process(text, question):
sentence_tokens = nltk.sent_tokenize(text)
sentence_tokens.append(question)
tv = TfidfVectorizer(tokenizer=lemma_me)
tf = tv.fit_transform(sentence_tokens)
values = cosine_similarity(tf[-1], tf)
index = values.argsort()[0][-2]
values_flat = values.flatten()
values_flat.sort()
coeff = values_flat[-2]
if coeff > 0.3:
return sentence_tokens[index]
while True:
question = input("Hi, what would you like to know about women ?\n")
output = process(text, question)
if output:
print(output)
elif question=='quit':
break
else:
print("Have no idea )))")
OUT: Hi, what would you like to know about women ?
who are women ?
A woman is an adult female human.
Hi, what would you like to know about women ?
how many women in population ?
Out of the total human population in 2015, there were 101.8 men for every 100 women.
Hi, what would you like to know about women ?