Handling the text.
This section explores a number of handy use cases that usually arise when processing text with Python.
By text files here we usually mean non-binary files containing text information without additional format info - .txt, .csv, .py, .html, etc.
Processing text files in Python is relatively easy to compare with most of the other programming languages.
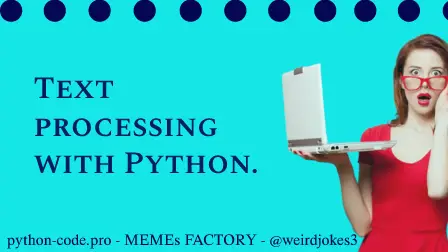
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-03 by Andrey BRATUS, Senior Data Analyst.
Reading a textfile:
Creating new text file with your content:
Removing last character from text file:
Removing last character in several files:
Replace a word in several files:
Merging text from several files:
Replacing text line in txt or csv file:
In Python, there is no need for importing external libraries to read and write files. Python provides an inbuilt function for creating, writing, and reading files. Usually, we just use the “open()” function with reading or writing mode and then start to loop the text files line by line? which is even better using WITH context manager.
with open('myfile.txt', 'r') as file:
content = file.read()
print(content)
OUT: I am a file content.
content = """Welcome to
www.python-code.pro"""
with open('myfile1.txt', 'w') as file:
file.write(content)
print('My text added to the file !!!')
OUT: My text added to the file !!!
with open('file.csv', 'r') as file:
content = file.read()
modified_content = content[:-1]
with open('new-file.csv', 'w') as file:
file.write(modified_content)
print('new file without last character was created !!!')
OUT: new file without last character was created !!!
from pathlib import Path
files_dir = Path('files')
for filepath in files_dir.iterdir():
with open(filepath, 'r') as file:
content = file.read()
new_content = content[:-1]
with open(filepath, 'w') as file:
file.write(new_content)
print('last character was successfully removed !!!')
OUT: last character was successfully removed !!!
from pathlib import Path
files_dir = Path('myfiles')
for filepath in files_dir.iterdir():
with open(filepath, 'r') as file:
content = file.read()
new_content = content.replace('word', 'phrase')
with open(filepath, 'w') as file:
file.write(new_content)
print('word is replaced !!!')
OUT: word is replaced !!!
from pathlib import Path
files_dir = Path('myfiles')
merged = ''
for filepath in files_dir.iterdir():
with open(filepath, 'r') as file:
content = file.read()
merged = merged + content + '\n'
with open('result.csv', 'w') as file:
file.write(merged)
print('files are merged in a result file !!!')
OUT: files are merged in a result file !!!
with open('original.csv', 'r') as file:
content = file.readlines()
content[0] = 'sex,drugs,rocknroll\n'
with open('new.csv', 'w') as file:
file.writelines(content)
print('text line was replaced !!!')
OUT: text line was replaced !!!