Handlig video files.
As for earlier described image processing here the video processing also can be performed using Open Source Computer Vision Library (OpenCV). which is a great choice to perform computationally intensive computer vision tasks and used in the following examples.
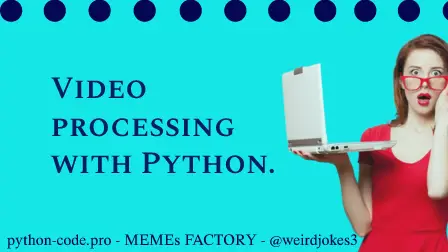
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Getting video information with OpenCV:
Extracting image frames from video with OpenCV:
Extracting video frame at specific timestamp:
Hilighting faces in a video:
Capture, play, detect faces and save a video from a webcam:
Usually a video processing is performing operations on the video frame by frame. Frames are just the instances of the video in a single point of time. So every operations performed on images can be performed on frames as well.
import cv2
video = cv2.VideoCapture("my_video.mp4")
width = int(video.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(video.get(cv2.CAP_PROP_FRAME_HEIGHT))
nr_frames = int(video.get(cv2.CAP_PROP_FRAME_COUNT))
fps = int(video.get(cv2.CAP_PROP_FPS))
print(f"width ={width}, height ={height}, number of frames ={nr_frames},frames per second ={fps}")
OUT: width =1920, height =1080, number of frames =90,frames per second =30
import cv2
video = cv2.VideoCapture("video.mp4")
success, frame = video.read()
count = 1
while success:
cv2.imwrite(f'images/im-{count}.jpg', frame)
success, frame = video.read()
count += 1
print('all frames are extracted !!!')
OUT: all frames are extracted !!!
import cv2
video = cv2.VideoCapture("video.mp4")
nr_frames = video.get(cv2.CAP_PROP_FRAME_COUNT)
fps = video.get(cv2.CAP_PROP_FPS)
timestamp = input('Enter timestamp in hh:mm:ss: ')
timestamp_list = timestamp.split(':')
hh, mm, ss = timestamp_list
timestamp_list_floats = [float(i) for i in timestamp_list]
hours, minutes, seconds = timestamp_list_floats
frame_nr = hours * 3600 * fps + minutes * 60 * fps + seconds * fps
video.set(1, frame_nr)
success, frame = video.read()
cv2.imwrite(f'Frame -{hh}:{mm}:{ss}.jpg', frame)
print('frame is extracted !!!')
OUT: frame is extracted !!!
import cv2
video = cv2.VideoCapture("sourcevideo.mp4")
success, frame = video.read()
height = frame.shape[0]
width = frame.shape[1]
#you will need faces.xml cascade classifier for face detection
face_cascade = cv2.CascadeClassifier('faces.xml')
output = cv2.VideoWriter('result_video.avi',
cv2.VideoWriter_fourcc(*'DIVX'), 30, (width, height))
# count = 0
while success:
faces = face_cascade.detectMultiScale(frame, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 255, 255), 4)
output.write(frame)
success, frame = video.read()
# count += 1
# print(count)
output.release()
print("Faces are hilighted in result file !!!")
OUT: Faces are hilighted in result file !!!
The following script captures video from a webcam, detects faces in it, plays the result online and saves the result video on your hard drive.
You will need faces.xml cascade classifier for face detection.
Press 'q' to quit the program !!!
import cv2
video = cv2.VideoCapture(0)
success, frame = video.read()
height = frame.shape[0]
width = frame.shape[1]
#you will need faces.xml cascade classifier for face detection
face_cascade = cv2.CascadeClassifier('faces.xml')
output = cv2.VideoWriter('result_video.avi',
cv2.VideoWriter_fourcc(*'DIVX'), 15, (width, height))
# count = 0
while success:
faces = face_cascade.detectMultiScale(frame, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 255, 255), 4)
cv2.imshow("Recording", frame)
key=cv2.waitKey(1)
# press q to quit the program
if key==ord('q'):
break
output.write(frame)
success, frame = video.read()
# count += 1
# print(count)
output.release()
video.release()
cv2.destroyAllWindows()
print("Faces are hilighted in а result file !!!")
OUT: Faces are hilighted in а result file !!!