Files upload / download.
The Python 'Requests' library is a neat, user-friendly HTTP module and one of the most popular packages as it's widely used for downloading and uploading files on the web. The Requests package isn’t part of Python’s standard library at least at the moment and should be installed by pip install requests.
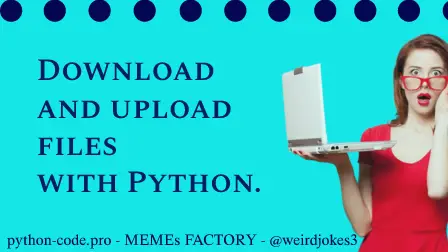
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-11 by Andrey BRATUS, Senior Data Analyst.
Downloading MP3 file from Internet:
Uploading text file on the free web server:
Two simple but still useful use cases below show examples of downloading MP3 file from the WEB and uploading text file on the free server.
import requests
url = "https://filesamples.com/samples/audio/mp3/sample1.mp3"
req = requests.get(url)
content = req.content
with open('sample1.mp3', 'wb') as file:
file.write(content)
print('MP3 file is downloaded !!!')
OUT: MP3 file is downloaded !!!
import requests
url = "https://cgi-lib.berkeley.edu/ex/fup.cgi"
file = open('textfile.txt', 'rb')
req = requests.post(url, files={"upfile":file})
#You can display HTML response
# print(req.text)
print("You've uploaded a file.")
OUT: You've uploaded a file.