Image handling.
The knowledge and understanding of this world comes from data analysis and images are a substantial part of this data. Before they can be used, these digital images must be processed and played around in order to improve their visual quality and extract some information that can be put to use.
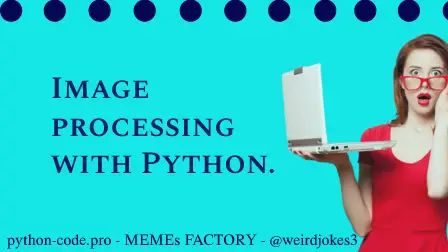
Python is a cool and modern choice for these types of image processing tasks due to its growing popularity as a scientific
programming language and the free availability of many recent
image processing libraries in its ecosystem.
Open Source Computer Vision Library or simply OpenCV is one of the most widely used libraries for computer vision applications.
It is a great choice to perform computationally intensive computer vision tasks and used in the following examples.
Convert single image to grayscale with OpenCV:
import cv2
color = cv2.imread('bratusnet.jpeg', 0)
cv2.imwrite('bratusnet-gray.jpeg', color)
print('image converted')
OUT: image converted
Convert several images to grayscale with OpenCV:
import os
import cv2
images = os.listdir('img')
for image in images:
# print(image)
gray = cv2.imread(f'img/{image}', 0)
# print(gray)
cv2.imwrite(f'grayscaled/gray-{image}', gray)
print('images are converted')
OUT: images are converted
Image resize with OpenCV:
import cv2
#scale=1/10
def calculate_size(scale_percentage, width, height):
new_width = int(width * scale_percentage / 10)
new_height = int(height * scale_percentage / 10)
print("New Dimensions:", new_width, new_height)
return (new_width, new_height)
def resize(image_path, scale_percentage, resized_path):
image = cv2.imread(image_path)
new_dim = calculate_size(scale_percentage, image.shape[1], image.shape[0])
resized_image = cv2.resize(image, new_dim)
cv2.imwrite(resized_path, resized_image)
resize('bratusnet.jpeg', 10, 'resized-bratusnet.jpeg')
print("Image resized")
OUT: New Dimensions: 438 779
Image resized
Several images resize with OpenCV:
import cv2
import os
#scale=1/10
def calculate_size(scale_percentage, width, height):
new_width = int(width * scale_percentage / 10)
new_height = int(height * scale_percentage / 10)
#print("New Dimensions:", new_width, new_height)
return (new_width, new_height)
def resize(image_path, scale_percentage, resized_path):
image = cv2.imread(image_path)
new_dim = calculate_size(scale_percentage, image.shape[1], image.shape[0])
resized_image = cv2.resize(image, new_dim)
cv2.imwrite(resized_path, resized_image)
images = os.listdir('images')
for image in images:
resize(f'images/{image}', 10, f'resized/resized-{image}')
print("Images are resized")
OUT: Images are resized
Detect faces in images with OpenCV:
import cv2
#you will need faces.xml cascade classifier for face detection
image = cv2.imread('humans.jpeg', 1)
face_cascade = cv2.CascadeClassifier('faces.xml')
faces = face_cascade.detectMultiScale(image, 1.1, 4)
# print(faces)
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 255, 255), 4)
cv2.imwrite('faces_detected.jpeg', image)
print('image with faces created')
OUT: image with faces created
Changing background:
The background of original image should be monochrome or approximately the same color.
import cv2
import numpy as np
foreground = cv2.imread("source.jpeg")
background = cv2.imread("back.jpeg")
# print(foreground[40, 40])
width = foreground.shape[1]
height = foreground.shape[0]
# print(foreground.shape)
resized_background = cv2.resize(background, (width, height))
for i in range(width):
for j in range(height):
pixel = foreground[j, i]
# print(type(pixel))
if np.any(pixel == [1, 255, 0]):
foreground[j, i] = resized_background[j, i]
cv2.imwrite('output.jpeg', foreground)
print('background added !!!')
OUT: background added !!!
Putting watermark on original image:
import cv2
# Load the two images
image = cv2.imread('original.jpeg')
watermark = cv2.imread('watermark.png')
# print(watermark.shape)
x = image.shape[1] - watermark.shape[1]
y = image.shape[0] - watermark.shape[0]
watermark_place = image[y:, x:]
cv2.imwrite('watermark_place.jpeg', watermark_place)
# print(watermark_place.shape)
blend = cv2.addWeighted(watermark_place, 0.5, watermark, 0.5, 0)
cv2.imwrite('mix.jpeg', blend)
image[y:, x:] = blend
cv2.imwrite('origin-watermark.jpeg', image)
print('watermark added !!!')
OUT: watermark added !!!