Generating password.
Password generation is a handy usecase of Python application. The code below asks user to provide password parameters such as number of letters that should be used, how many numbers and special symbols should be implemented.
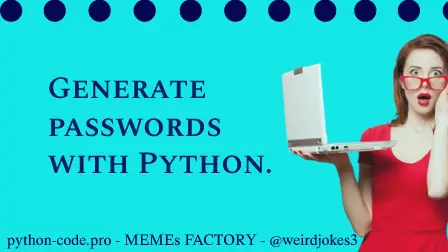
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-07 by Andrey BRATUS, Senior Data Analyst.
If in your case no user input is required, just fix this variables at the beginning of your code. The result will contain generated password with random uppercase/lowercase letters, numbers and symbols in random order. Thats it !!!
Password generator Python code:
import random
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
numbers = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']
symbols = ['!', '#', '$', '%', '&', '(', ')', '*', '+']
print("Welcome to the My Password Generator!")
nr_letters = int(input("How many letters would you like in new password?\n"))
nr_symbols = int(input(f"How many symbols would you like?\n"))
nr_numbers = int(input(f"How many numbers would you like?\n"))
password_list = []
for char in range(1, nr_letters + 1):
password_list.append(random.choice(letters))
for char in range(1, nr_symbols + 1):
password_list += random.choice(symbols)
for char in range(1, nr_numbers + 1):
password_list += random.choice(numbers)
# print(password_list)
random.shuffle(password_list)
# print(password_list)
password = ""
for char in password_list:
password += char
print(f"New password is: {password}")
OUT: Welcome to the My Password Generator!
How many letters would you like in new password?
3
How many symbols would you like?
2
How many numbers would you like?
5
New password is: p3!82)23hg