Drawing polygons.
To draw Voronoi polygons in Python is an easy task using Scipy library, but if you will face the task to find initial dots coordinates and their corresponding polygon coordinates and try to find ready solution in internet most probably you will fail.
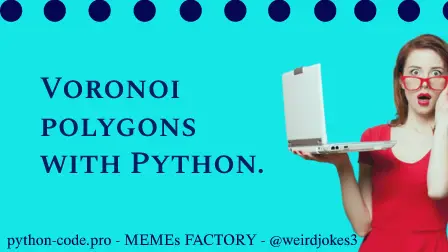
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
So i share my solution that probably will help.
#Importing the libraries
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
from scipy.spatial import Voronoi, voronoi_plot_2d
#Importing the dataset
sites=pd.read_excel('coordinates.xlsx')
y = sites [['LATITUDE', 'LONGITUDE']]
#Drawing polygons
vor = Voronoi(y)
fig = voronoi_plot_2d(vor)
plt,show()
#getting sites coordinates for all polygons
dfp3 =pd.DataFrame(columns=['REGION'] )
RNG2=len(vor.points)-1
for i in range(0,RNG2):
dfp = pd.DataFrame([vor.point_region[i]],columns=['REGION'])
dfp.insert(1,'LATITUDE',vor.points[i][0])
dfp.insert(2,'LONGITUDE',vor.points[i][1])
dfp2=dfp
frames = [dfp3, dfp2]
dfp3 = pd.concat(frames)
dfp3
# Getting polygons GEO coordinates
# Correcting GEO coordinates if needed - depends of the task you solve, maybe you can skip correction step
RNG=len(vor.regions)-1
df3 =pd.DataFrame(columns=['VORONOY'] )
df2 =pd.DataFrame(columns=['REGION', 'VORONOY'] )
for i in range(0,RNG):
RNG1=len(vor.regions[i])
if RNG1>0 and (-1 not in vor.regions[i]):
s=''
for k in range(0,RNG1):
lo=vor.vertices[vor.regions[i]][k][1]
lon =lo if abs(lo)<180.00000000000000 else 180.00000000000000
la=vor.vertices[vor.regions[i]][k][0]
lat =la if abs(la)<90.00000000000000 else 90.00000000000000
s1=str(lon)+' '+str(lat)
s=s+','+s1
lo1=vor.vertices[vor.regions[i]][0][1]
lon1 =lo1 if abs(lo1)<180.00000000000000 else 180.00000000000000
la1=vor.vertices[vor.regions[i]][0][0]
lat1 =la1 if abs(la1)<90.00000000000000 else 90.00000000000000
s2=str(lon1)+' '+str(lat1)
s3=s+','+s2
s3=s3[1:]
df = pd.DataFrame([i],columns=['REGION'])
df.insert(1,'VORONOY',s3)
df2=df
else:
pass
frames = [df3, df2]
df3 = pd.concat(frames)
# Joining sites to polygons
df3 = df3.drop_duplicates(subset=['VORONOY'])
result1 = pd.merge(dfp3, df3, on=["REGION"])
result = pd.merge(sites, result1, on=["LATITUDE", "LONGITUDE"])
result = result.drop_duplicates(subset=['PLACE_NAME'])
# result = result.reset_index()
result
#Exporting resulting table as XLSX
result.to_excel('voronoi_results.xlsx')