Manipulating PC devices.
Python is a modern powerful programming language that lets you write code quickly and effectively. Thanks to the rich availability of additional libraries, Python can now be used to control PC hardware like webcams, microfone, mouse and keybord, network devices, etc.
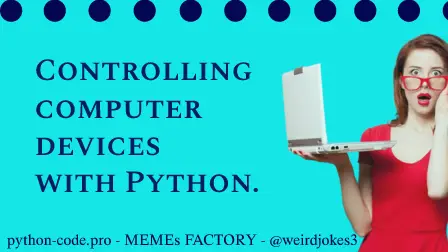
For taking screenshots you can use MSS (Multiple Screen Shots) library - an ultra fast cross-platform multiple screenshots module in pure python using ctypes.
For microphone usage you can use sounddevice library - a Python module providing bindings for the PortAudio library and a few convenience functions to play and record NumPy arrays containing audio signals.
For mouse and keyboard control you can use pyautogui library that lets your Python scripts control the mouse and keyboard to automate interactions with other applications.
Taking screenshots from your PC:
IMPORTANT: first you need to install mss library by 'conda install -c conda-forge python-mss' or 'pip install mss'.
from mss import mss
with mss() as screen:
screen.shot(output='screenshot1.png')
print('Your screenshot is saved !!!')
OUT: Your screenshot is saved !!!
Recording MP3 file from your microphone:
IMPORTANT: first you need to install sounddevice library by 'pip install sounddevice'.
import sounddevice
from scipy.io.wavfile import write
seconds = 8 #duration
fps = 44100 #sampling rate in HERTZ
myrecording = sounddevice.rec(frames=seconds*fps, samplerate=fps, channels=1)
sounddevice.wait()
write('my.mp3', fps, myrecording)
print('New mp3 was created !!!')
OUT: New mp3 was created !!!
PC mouse control with Python:
IMPORTANT: first you need to install sounddevice library by 'pip install pyautogui'.
import pyautogui
pyautogui.click(100,100, clicks=10,interval=0.5) # Performs pressing a mouse button down and then immediately releasing it.
pyautogui.moveTo(500, 500) #Moves the mouse cursor to a point on the screen.
print("Just clicked 10 times and moved to 500, 500.")
OUT: Just clicked 10 times and moved to 500, 500.