Handling your emails.
Sending emails in everyday life is quite an easy task using email applications or web interface. But sometimes you need to automate your routine tasks and distribute information right from the place where it was generated. Thats where Python should be used !!!
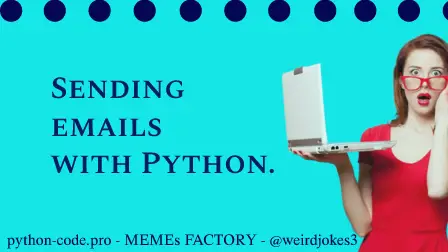
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Sending single e-mail with Python:
Sending an e-mail periodically with Python:
Sending an e-mail at a certain time with Python:
Sending an e-mail to contact list (in CSV):
Sending an e-mail with attachment:
Simple but efficient scripts below are ready to help, just substitute example data with your own.
IMPORTANT!!!: you can specify password information in explicit way, but it's not recommended for security reasons. You can also store password in external file, tnen import it in a script and use or store it in environment as shown below.
import yagmail
import os
sender = 'my_example@gmail.com'
receiver = 'my_example@python-code.pro'
subject = "Crazy example subject!"
contents = """
For best jokes on the web visit https://python-code.pro !!!
"""
yag = yagmail.SMTP(user=sender, password=os.getenv('PASSWORD'))
yag.send(to=receiver, subject=subject, contents=contents)
print("Crazy Email Sent!")
OUT: Crazy Email Sent!
import yagmail
import os
import time
sender = 'my_example@gmail.com'
receiver = 'my_example@python-code.pro'
subject = "Crazy example subject!"
contents = """
For best jokes on the web visit https://python-code.pro !!!
"""
while True:
yag = yagmail.SMTP(user=sender, password=os.getenv('PASSWORD'))
yag.send(to=receiver, subject=subject, contents=contents)
print("Crazy Email Sent!")
time.sleep(60)
OUT: Crazy Email Sent!
import yagmail
import os
import time
from datetime import datetime as dt
sender = 'my_example@gmail.com'
receiver = 'my_example@python-code.pro'
subject = "Crazy example subject!"
contents = """
For best jokes on the web visit https://python-code.pro !!!
"""
while True:
now = dt.now()
if now.hour == 23 and now.minute == 59:
yag = yagmail.SMTP(user=sender, password=os.getenv('PASSWORD'))
yag.send(to=receiver, subject=subject, contents=contents)
print("Crazy Email Sent!")
time.sleep(60)
OUT: Crazy Email Sent!
import yagmail
import os
import pandas
sender = 'my_example@gmail.com'
subject = "Crazy example subject!"
yag = yagmail.SMTP(user=sender, password=os.getenv('PASSWORD'))
df = pandas.read_csv('my_contacts.csv')
for index, row in df.iterrows():
contents = f"""
Hi {row['name']} .For best jokes on the web visit https://python-code.pro !!!
"""
yag.send(to=row['email'], subject=subject, contents=contents)
print("Crazy Email Sent!")
OUT: Crazy Email Sent!
Crazy Email Sent!
Crazy Email Sent!
import yagmail
import os
sender = 'my_example@gmail.com'
receiver = 'my_example@python-code.pro'
subject = "Crazy example subject!"
contents = ["""
For best jokes on the web visit https://python-code.pro !!!
""", 'attachment.txt']
yag = yagmail.SMTP(user=sender, password=os.getenv('PASSWORD'))
yag.send(to=receiver, subject=subject, contents=contents)
print("Crazy Email Sent!")
OUT: Crazy Email Sent!