Weather forecast updates.
Let's proceed our BeautifulSoup experiments and after HTML table scrapping we will try another usecase - finding current weather from Google site.
Let me remind you that BeautifulSoup is a Python library used to extract necessary data from HTML and XML files i.e for web scraping purposes.
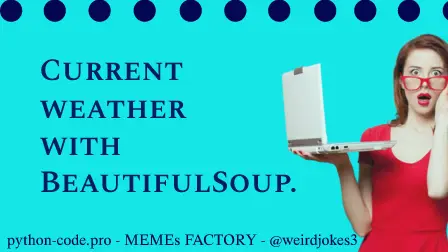
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-11 by Andrey BRATUS, Senior Data Analyst.
It produces a parse tree from the page source code, which can be used to extract data in a more readable/hierarchical way.
Let's start from importing bs4 and requests, most probably you wll need to install them first in your environment. Here we will use Python’s requests module to make HTTP requests
import requests
from bs4 import BeautifulSoup
We will need headers because the headers contain protocol-specific information that is placed before the raw message i.e retrieved from the website.
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
Now we need to define the Python function which does all the job.
def weather(city):
city = city.replace(" ", "+")
res = requests.get(
f'https://www.google.com/search?q={city}&oq={city}&aqs=chrome.0.35i39l2j0l4j46j69i60.6128j1j7&sourceid=chrome&ie=UTF-8', headers=headers)
print("Searching for weather info...\n")
soup = BeautifulSoup(res.text, 'html.parser')
location = soup.select('#wob_loc')[0].getText().strip()
time = soup.select('#wob_dts')[0].getText().strip()
info = soup.select('#wob_dc')[0].getText().strip()
weather = soup.select('#wob_tm')[0].getText().strip()
print(location)
print(time)
print(info)
print(weather+"°C")
And finally we ask the user the name of desired city, use our function and providing all the results.
city = input("Enter the Name of your City -> ")
city = city+" weather"
weather(city)
print("Have a Nice Day from python-code.pro :)")
OUT:
Enter the Name of your City -> London
Searching for weather info...
London, UK
Sunday 2:00 PM
Partly cloudy
14°C
Have a Nice Day from python-code.pro :)