Handling files and folders.
Python is one of the best modern programming languages to automate the regular system management stuff and has several built-in tools or modules for handling files and folders operations. The most common and traditional way for these operations is to use os library, but pathlib is more modern and flexible library for such tasks.
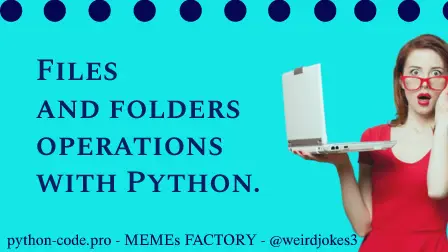
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
The pathlib is a Python module which provides an object API for working with files and directories. This module offers classes representing filesystem paths with semantics appropriate for different operating systems. The pathlib is a standard module? you don't need to install it.
Files search in your competer:
Creating a bunch of empty files:
Deleting the files:
Renaming all files in a folder (add prefix):
Renaming files using folder name:
Adding file created dates to filenames in a folder:
Changing files extensions to all files in a folder:
Creating zip archive for files:
Unzip the files:
from pathlib import Path
root_dir = Path('.')
search_term = 'bratus'
for path in root_dir.rglob("*"):
if path.is_file():#remove this if if you also need folders search
if search_term in path.stem:
print(path.absolute())
OUT: /home/andrey/bratus.html
from pathlib import Path
root_dir = Path('newfiles')
for i in range(1, 24):
filename = str(i) + '.csv'
filepath = root_dir / Path(filename)
filepath.touch()
print('files were created !!!')
OUT: files were created !!!
from pathlib import Path
root_dir = Path('destination')
for path in root_dir.glob("*.html"):
with open(path, 'wb') as file:
file.write(b'')#empties the file
path.unlink()#deletes the file
print("files are deleted completely !!!")
OUT: files are deleted completely !!!
from pathlib import Path
root_dir = Path('filesfolder')
file_paths = root_dir.iterdir()
# print(Path.cwd())
for path in file_paths:
new_filename = "my-" + path.stem + path.suffix
new_filepath = path.with_name(new_filename)
# print(new_filepath)
path.rename(new_filepath)
print('All files were renamed !!!')
OUT: All files were renamed !!!
from pathlib import Path
root_dir = Path('myfiles')
file_paths = root_dir.glob("**/*")
for path in file_paths:
if path.is_file():
parent_folder = path.parts[-2]
new_filename = parent_folder + '-' + path.name
# print(new_filename)
new_filepath = path.with_name(new_filename)
path.rename(new_filepath)
print('All files were renamed !!!')
OUT: All files were renamed !!!
from pathlib import Path
from datetime import datetime
root_dir = Path('myfiles')
for path in root_dir.glob("**/*"):
if path.is_file():
created_date = datetime.fromtimestamp(path.stat().st_ctime)
created_date_str = created_date.strftime("%Y-%m-%d_%H:%M:%S")
new_filename = created_date_str + '_' + path.name
new_filepath = path.with_name(new_filename)
path.rename(new_filepath)
print('File created dates were added to filenames !!!')
OUT: File created dates were added to filenames !!!
from pathlib import Path
root_dir = Path('myfiles')
for path in root_dir.rglob("*.csv"):
if path.is_file():
new_filepath = path.with_suffix(".txt")
path.rename(new_filepath)
print('Files extensions were changed !!!')
OUT: Files extensions were changed !!!
from pathlib import Path
import zipfile
root_dir = Path('files')
archive_path = root_dir / Path('myarchive.zip')
with zipfile.ZipFile(archive_path, 'w') as zf:
for path in root_dir.rglob("*.csv"):
zf.write(path)
# unlink if original files to be removed
# path.unlink()
print('Archive is created !!!')
OUT: Archive is created !!!
from pathlib import Path
import zipfile
root_dir = Path('.')
destination_path = Path('destination')
for path in root_dir.glob("*.zip"):
with zipfile.ZipFile(path, 'r') as zf:
final_path = destination_path / Path(path.stem)
zf.extractall(path=final_path)
print('Files are unzipped !!!')
OUT: Files are unzipped !!!