Maximizing SEO.
Unlock the power of data-driven insights with Python and Yandex Webmaster API. As the digital landscape evolves, mastering the art of SEO becomes increasingly crucial. Our comprehensive guide will walk you through the intricacies of leveraging Yandex's robust Webmaster API using Python, the world's most popular programming language. Whether you're a seasoned developer or a digital marketing enthusiast, this page will serve as your roadmap to enhancing your website's visibility, driving organic traffic, and ultimately, achieving your business goals.
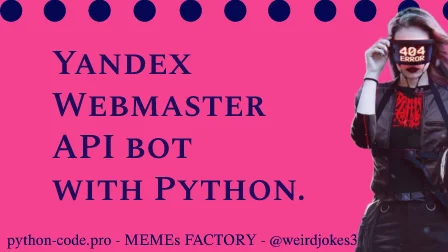
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Getting Started with Yandex Webmaster API.
Using Yandex Webmaster API with Python.
Benefits of using Yandex Webmaster API with Python.
Common errors and their solutions.
Conclusion.
Let's dive into the world where Python meets Yandex, and SEO success is just a few lines of code away.
Yandex search engine provides very powerfull Webmaster online web interface, but you can get most of it's useful information using Yandex Webmaster API and the power of Python. Then you can transform webmaster data in any easy-to-read format for postprocessing and visualisation.
You will need the same ACCESS_TOKEN, that we got in previous section about Yandex Metrika.
Getting started with Yandex Webmaster API is not as difficult as it might seem. The first step is to create a Yandex Webmaster account, which is a quick and simple process. Once you have created your account, you can proceed to get API access, and for this step, you will need to follow the instructions provided by Yandex Webmaster.
After you have received API access, you will need to install the required Python packages. Installing the packages is easy, and the process is well-documented on the official Python documentation page. The final step is to create a Python script that will use the Yandex Webmaster API to retrieve the data you need.
It might seem daunting to create a Python script, but Yandex has provided detailed guidance on how to create the script. By following the instructions, you will be able to create your script in no time. Keep in mind that while creating your Python script, you might face some errors, but Yandex provides a wealth of information on how to fix them.
With these key steps, you will be well on your way to using Yandex Webmaster API with Python. And the best part is that once you have set up everything, you will be able to automate data retrieval and analysis, save time and effort, and create customized reporting options easily.
Are you tired of manually retrieving and analyzing data from Yandex Webmaster? Well, worry no more! With the Yandex Webmaster API and Python, you can get your hands on insightful data without breaking a sweat.
First things first, to use the API, you need to authorize your account. Once you have access, you can start retrieving important site information such as crawl errors, indexed pages, and crawl statistics. But that's not all! You can also retrieve search queries and keywords to gain insights into your audience's search behavior.
And let's not forget about backlink data. With the API, you can easily retrieve data on the number and quality of backlinks to your site. This information is crucial for improving your overall SEO strategy.
Oh, and did we mention updating your sitemap through the API? Yep, it's possible, saving you time and effort in the process.
The benefits of using the Yandex Webmaster API with Python are endless. With automated data retrieval and customized reporting options, you'll be saving time and making informed decisions in no time.
Don't let common errors like authorization, API or Python script related issues hold you back. With a little troubleshooting, you'll be able to use the API and Python to your advantage in no time.
Get started with the Yandex Webmaster API and Python today and take your site's SEO to the next level!
Lets start from installing Yandex webmaster API library.
pip install yandex-webmaster-api
Then we should create client instance with your ACCESS_TOKEN.
from yandex_webmaster import YandexWebmaster
client = YandexWebmaster('')
Now we are ready to check available hosts for our credentials, we will need host IDs for the next steps.
hosts = client.get_hosts()
hosts
OUT:
[{'ascii_host_url': 'https://python-code.pro/',
'host_id': 'https:python-code.pro:443',
'main_mirror': None,
'unicode_host_url': 'https://python-code.pro/',
'verified': True},
{'ascii_host_url': 'https://weird-jokes.com/',
'host_id': 'https:weird-jokes.com:443',
'main_mirror': None,
'unicode_host_url': 'https://weird-jokes.com/',
'verified': True}]
Then let's check if certain site is indexed and available for search.
result1 = client.get_host('https:python-code.pro:443')
result1
OUT:
{'ascii_host_url': 'https://python-code.pro/',
'host_data_status': 'OK',
'host_display_name': 'python-code.pro',
'host_id': 'https:python-code.pro:443',
'main_mirror': None,
'unicode_host_url': 'https://python-code.pro/',
'verified': True}
Now we are ready to display popular search queries for you site setting time period.
from datetime import datetime, timedelta
date_from = datetime.now() - timedelta(days=30)
date_to = datetime.now()
result2 = client.get_popular_search_queries('https:python-code.pro:443', date_from, date_to, query_indicator='TOTAL_SHOWS')
result2
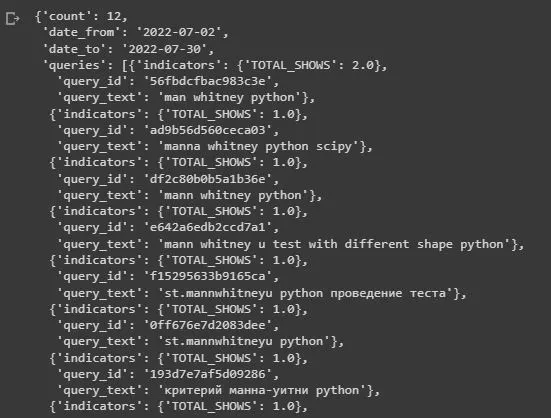
Then let's check status and information about our sitemap file.
result3 = client.get_sitemaps(host_id='https:python-code.pro:443')
result3
OUT:
{'sitemaps': [{'children_count': 0,
'errors_count': 0,
'last_access_date': '2022-07-31T03:41:57.000+03:00',
'sitemap_id': '0500919e-ba70-32dd-a204-b970a136d873',
'sitemap_type': 'SITEMAP',
'sitemap_url': 'https://python-code.pro/static/img/sitemap.xml',
'sources': ['ROBOTS_TXT', 'WEBMASTER'],
'urls_count': 138}]}
Now let's see site's indexing statistics and check if there are some problems.
result4 = client.get_indexing_stats(host_id='https:weird-jokes.com:443')
result4
OUT:
{'excluded_pages_count': 0,
'searchable_pages_count': 316,
'site_problems': {},
'sqi': 0}
Checking for site problems known by Yandex.
result5 = client.diagnostic_site(host_id='https:python-code.pro:443')
result5
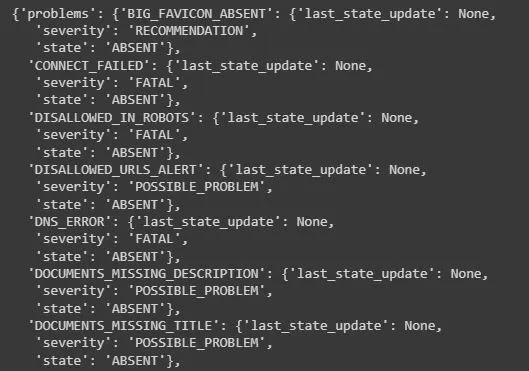
Check indexing information by pages.
result6 = client.get_indexing_samples(host_id='https:weird-jokes.com:443')
result6
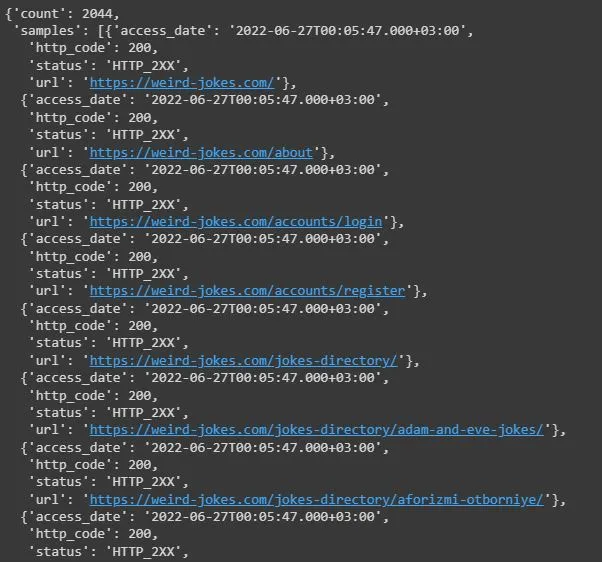
Using Yandex Webmaster API with Python has numerous benefits and can significantly enhance website management and analysis. Automated data retrieval and analysis saves time and effort, while customized reporting options allow for a personalized experience. Additionally, using Python can provide more flexibility than the standard Yandex Webmaster interface, enabling more detailed analysis. Overall, this combination of tools maximizes website optimization potential.
While using Yandex Webmaster API with Python, there may be some common errors that you could encounter. It could be related to authorization, API or Python script. One common error could be "Invalid authentication credentials" while trying to authorize the API. This error can be resolved by checking the accuracy of the credentials entered. Another error could be "Invalid response from API" which could arise due to server issues or invalid query parameters.
To avoid authorization related errors, ensure that the access token is accurate and the scope of access is appropriate. While dealing with API related errors, it is essential to verify the API documentation and the HTTP response codes to get an understanding of the issue. With regards to Python script related errors, it is essential to check for syntax errors, variable assignment, and code structure.
In case of any errors, the Yandex API documentation has detailed explanations of common errors and their solutions. It is also important to note that handling errors effectively requires prior understanding of the API functions.
If you're looking to streamline your site's data analysis and reporting, using Yandex Webmaster API with Python is the way to go. With automated retrieval of site information, search queries and keyword analysis, and custom reporting options, you'll save valuable time and effort. Plus, updating sitemap is a breeze. Don't be left behind in the digital race. Join the trend and try it out for yourselves!