Mobile friendliness examination.
If you are a webmaster one of the tasks you solve is to provide that your website's pages are equaly adapted for desktop and mobile views. Google APIs and Python can be used to check if your webpages are mobile friendly, so for the script below you will need to get your own API key, please don't try to use the key from the script as it was changed for security reasons.
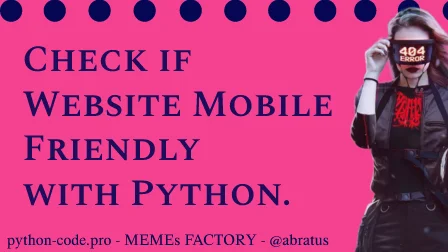
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-14 by Andrey BRATUS, Senior Data Analyst.
The logic of the Python code below is really simple:
- First you need pip install advertools which will convert your sitemap to dataframe.
- Get your API key from Google and use it in the script.
- Then import all necessary libraries.
- Convert sitemap to DF and then to list.
- Slice the list if you want to check just part of your website, e.g. just first 100 URLs.
- Using FOR loop check the mobile friendliness of each URL, see the results.
- You may need to adjust sleep time between each iteration, 20 worked perfect for me.
Check if Website is Mobile Friendly Python code:
import advertools as adv
import pandas as pd
import urllib.request
import time
import json
sitemap_urls = adv.sitemap_to_df("https://python-code.pro/static/img/sitemap.xml")
url = sitemap_urls["loc"].to_list()
url=url[5:8]
api_key = 'AIzaSyBgfdrsGnxrcVSrRowrhNvPECIYQfoDD-AMzwU'
service_url = 'https://searchconsole.googleapis.com/v1/urlTestingTools/mobileFriendlyTest:run'
for i in url:
status_code = urllib.request.urlopen(i).getcode()
print(i)
params = {
'url': i,
'key': api_key,
}
data = bytes(urllib.parse.urlencode(params), encoding='utf-8')
content = urllib.request.urlopen(url=service_url, data=data).read()
content_j=json.loads(content)
teststatus=content_j['testStatus']
friendly=content_j['mobileFriendliness']
print(f'{teststatus} {friendly}')
time.sleep(20)
print(f'Totally {len(url)} URLs checked.')
print('Thats all, folks !!!')
OUT:
INFO:root:Getting https://python-code.pro/static/img/sitemap.xml
https://python-code.pro/clustering-models-python-r-cheatsheets/
{'status': 'COMPLETE'} MOBILE_FRIENDLY
https://python-code.pro/association-rule-learning-python-r-cheatsheets/
{'status': 'COMPLETE'} MOBILE_FRIENDLY
https://python-code.pro/reinforcement-learning-python-r-cheatsheets/
{'status': 'COMPLETE'} MOBILE_FRIENDLY
Totally 3 URLs checked.
Thats all, folks !!!