Webpage speed examination.
In order to have good or at least acceptable ranking position in search engine results website owner should apply some measures to optimise page download performance.
When webpages are optimised, Python and Google PageSpeed Insights API are here to help for testing pages download performance with rich set of metrics.
For the webpage speed test script below you will need to get your own API key, please don't try to use the key from the script as it was changed for security reasons.
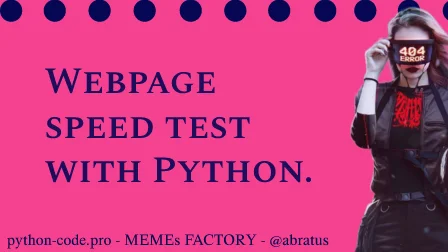
Python Knowledge Base: Make coding great again.
- Updated:
2024-10-20 by Andrey BRATUS, Senior Data Analyst.
The logic of the Python code below is really simple:
- Get your API key from Google and use it in the script.
- Then import all necessary libraries.
- Form the GET request using your API key and request URL to APIs endpoint.
- Postprocess and printout JSON from response.
- You can get additional information on metrics, their definitions and measurement units from the same JSON if you need.
- In case of addidional questions consult Google PageSpeed Insights API documentation.
Webpage speed test Python code:
import urllib.request
import json
api_key = 'AIzaSyBgfdrsGnxrcVSrRowrhNvPECIYQfoDD-AMzwU'
request_url = 'https://python-code.pro/'
url=f'https://www.googleapis.com/pagespeedonline/v5/runPagespeed?url={request_url}&key={api_key}'
response1 = urllib.request.urlopen(url).read()
response1_j=json.loads(response1)
target = response1_j['lighthouseResult']['requestedUrl']
metrics = response1_j['lighthouseResult']['audits']['metrics']['details']
print(f'Speed Test statistics for {target}')
metrics
Webpage speed test example output:
Speed Test statistics for https://python-code.pro/
{'items': [{'observedDomContentLoadedTs': 1695640886443,
'observedLoad': 6957,
'observedFirstContentfulPaintAllFrames': 3747,
'firstContentfulPaint': 730,
'observedFirstVisualChange': 3751,
'observedNavigationStartTs': 1695637020463,
'observedCumulativeLayoutShiftMainFrame': 0.0007992828451974433,
'observedLastVisualChangeTs': 1695642887463,
'observedFirstPaint': 3747,
'interactive': 1146,
'observedTraceEnd': 9981,
'observedFirstMeaningfulPaintTs': 1695640803494,
'observedLargestContentfulPaintAllFrames': 3783,
'totalBlockingTime': 41,
'observedLargestContentfulPaintTs': 1695640803494,
'observedCumulativeLayoutShift': 0.0007992828451974433,
'speedIndex': 2598,
'maxPotentialFID': 126,
'observedFirstContentfulPaintTs': 1695640767462,
'observedNavigationStart': 0,
'observedTimeOrigin': 0,
'observedFirstMeaningfulPaint': 3783,
'observedSpeedIndex': 3905,
'firstMeaningfulPaint': 730,
'observedFirstContentfulPaintAllFramesTs': 1695640767462,
'cumulativeLayoutShift': 0.0007992828451974433,
'observedLoadTs': 1695643977351,
'observedFirstVisualChangeTs': 1695640771463,
'cumulativeLayoutShiftMainFrame': 0.0007992828451974433,
'observedTraceEndTs': 1695647001379,
'observedFirstContentfulPaint': 3747,
'observedDomContentLoaded': 3866,
'observedSpeedIndexTs': 1695640925150,
'observedTimeOriginTs': 1695637020463,
'observedLargestContentfulPaintAllFramesTs': 1695640803494,
'observedLargestContentfulPaint': 3783,
'totalCumulativeLayoutShift': 0.0007992828451974433,
'largestContentfulPaint': 730,
'observedFirstPaintTs': 1695640767462,
'observedTotalCumulativeLayoutShift': 0.0007992828451974433,
'observedLastVisualChange': 5867},
{'lcpInvalidated': False}],
'type': 'debugdata'}