Getting small caps stocks data for trading.
Small-caps or shares of smaller companies is a special feild in investment, they are often attractive due to their lower relative valuations and potential to grow into big-cap stocks someday. Most investors stick only to big-caps and miss some potentially promising investment opportunities. According to Investopedia small-cap stocks generally have a market cap of $250 million to $2 billion.
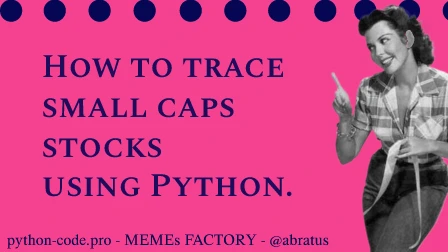
Python Knowledge Base: Make coding great again.
- Updated:
2025-07-10 by Andrey BRATUS, Senior Data Analyst.
Python code to get small caps gainers:
Small caps gainers output (shown partly):
Python code to get aggressive small caps:
Aggressive small caps output (shown partly):
In our case we will use Python to access trading statistics from Mboum Finance Official API which provides small caps stocks data.
To use necessary API you need to get "your-api-key" directly from Mboum Finance, or using rapidapi.com, the way I recommend.
Output data is in JSON format which can be easily converted to any suitable form for further visualisation and analysis,
small caps gainers data will surely help to catch market profits.
Small caps gainers: small Caps with a 1 day price change of 5.0% or more.
import requests
url = "https://mboum-finance.p.rapidapi.com/co/collections/small_cap_gainers"
querystring = {"start":"0"}
headers = {
"X-RapidAPI-Key": "your-api-key",
"X-RapidAPI-Host": "mboum-finance.p.rapidapi.com"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.text)
Aggressive small caps gainers: small cap stocks with earnings growth rates better than 25%.
import requests
url = "https://mboum-finance.p.rapidapi.com/co/collections/aggressive_small_caps"
querystring = {"start":"0"}
headers = {
"X-RapidAPI-Key": "your-api-key",
"X-RapidAPI-Host": "mboum-finance.p.rapidapi.com"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.text)