Network scanner use case.
Another simple example of Python usage is network scanner tool which will scan computers within the network and provide results to the user that can be used for network administration and ethical hacking purposes.
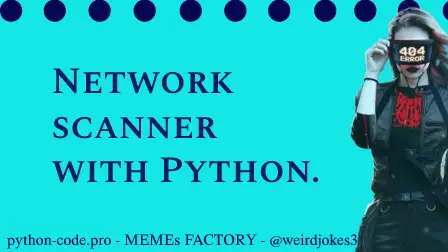
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Network scanner for security professionals:
Python code to implement Network scanner:
A Network Scanner is a Python-based tool utilized for ethical hacking that enables security professionals to discover active devices within a network and gather information about their configurations. By performing tasks such as ping sweeping, port scanning, and service detection, the scanner helps identify vulnerabilities and potential entry points for malicious attacks. This automated approach not only streamlines the reconnaissance phase of penetration testing but also assists organizations in strengthening their network security posture.
The code itself can be executed from command line specifying file name and IP address
- # python3 network_scanner.py --i 10.0.2.1/24 .
import scapy.all as scapy
import optparse
#function to deal with user input
def get_user_input():
parse_object = optparse.OptionParser()
parse_object.add_option("-i","--ipaddress", dest="ip_address",help="Enter IP Address")
(user_input,arguments) = parse_object.parse_args()
if not user_input.ip_address:
print("Enter IP Address")
return user_input
#function to make arp request, broadcasting it and dealing with response
def scan_my_network(ip):
arp_request_packet = scapy.ARP(pdst=ip)
#scapy.ls(scapy.ARP())
broadcast_packet = scapy.Ether(dst="ff:ff:ff:ff:ff:ff")
#scapy.ls(scapy.Ether())
combined_packet = broadcast_packet/arp_request_packet
(answered_list,unanswered_list) = scapy.srp(combined_packet,timeout=1)
answered_list.summary()
user_ip_address = get_user_input()
scan_my_network(user_ip_address.ip_address)