MAC changer use case.
As you might know Python is universal, quite simple and yet very powerful language thanks to variety of libraries wich support performing many different tasks. Below is an example of quite simple code wich will help you to change MAC address of desired interface in scope of your ethical hacking expiriments.
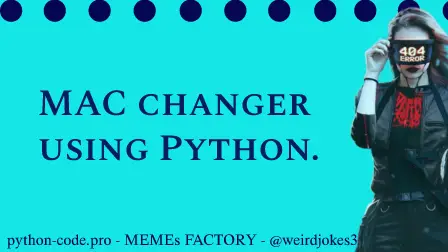
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Python is always at your service for ethical hacking purposes:
Python code to implement MAC changer:
The MAC Changer is a Python script designed for ethical hacking purposes that allows users to change their device's Media Access Control (MAC) address, enhancing privacy and anonymity while conducting security assessments. By manipulating the MAC address, ethical hackers can avoid tracking and maintain a level of stealth when testing network vulnerabilities. This tool is particularly beneficial for penetration testers seeking to simulate real-world attacks while protecting their identity and ensuring compliance with ethical guidelines.
The code itself can be executed from command line specifying file name, interface and new MAC address
- # python3 mac_changer.py --interface eth0 --mac 00-11-22-33-44-55 .
#importing the libraries
import subprocess
import optparse
import re
#function for getting user input
def get_user_input():
parse_object = optparse.OptionParser()
parse_object.add_option("-i","--interface",dest="interface",help="interface to change!")
parse_object.add_option("-m","--mac",dest="mac_address",help="new mac address")
return parse_object.parse_args()
#function for changing MAC
def change_mac_address(user_interface,user_mac_address):
subprocess.call(["ifconfig",user_interface,"down"])
subprocess.call(["ifconfig", user_interface,"hw","ether",user_mac_address])
subprocess.call(["ifconfig",user_interface,"up"])
#function for checking result
def control_new_mac(interface):
ifconfig = subprocess.check_output(["ifconfig",interface])
new_mac = re.search(r"\w\w:\w\w:\w\w:\w\w:\w\w:\w\w",str(ifconfig))
if new_mac:
return new_mac.group(0)
else:
return None
print("MyMacChanger started!")
(user_input,arguments) = get_user_input()
change_mac_address(user_input.interface,user_input.mac_address)
finalized_mac = control_new_mac(str(user_input.interface))
#feedback to the user
if finalized_mac == user_input.mac_address:
print("Your MAC was successfully canged!")
else:
print("Error - something went wrong!")